Summary for April 2025
Pixels and points, clicks and chills
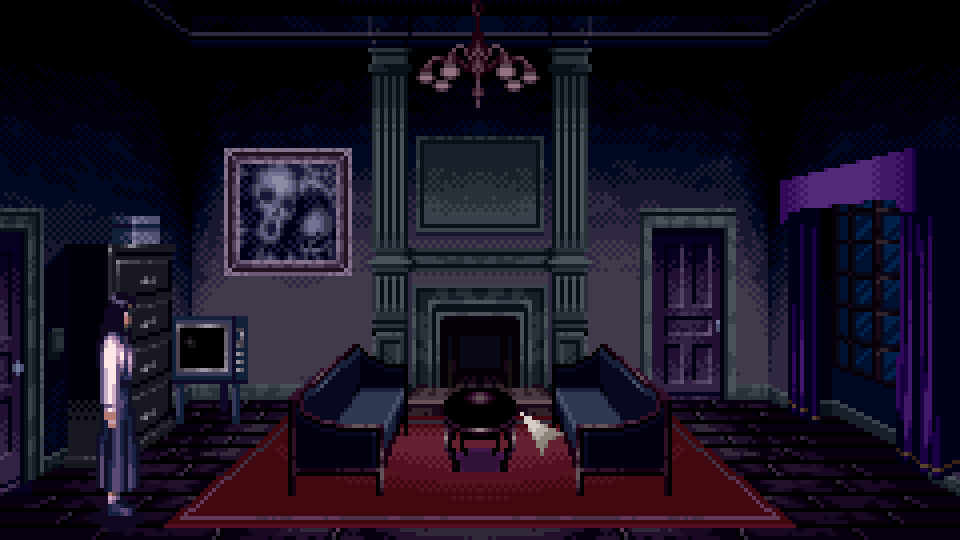
The Clock Tower series, and especially the first game, is often mentioned when it comes to horror video games. For good reason: you can clearly see how it became very influential, from the protagonist to the setting, from the puzzles to the atmosphere, from the visuals to the music (which, if not directly inspired, is very reminiscent of John Carpenter’s work).
What was a bit more surprising to me is how enjoyable it still is today, or at least Clock Tower: Rewind re-release. Which, to be fair, can be entirely down to me expecting some shenanigans from old games, when in fact they are often pretty straightforward. There are some frustrating parts of the point-and-click nature, but not many, and the potentially annoying mechanic of an enemy that can attack and chase you at any moment is not that big of a deal. Not flawless, there are some problematic things, but not many.
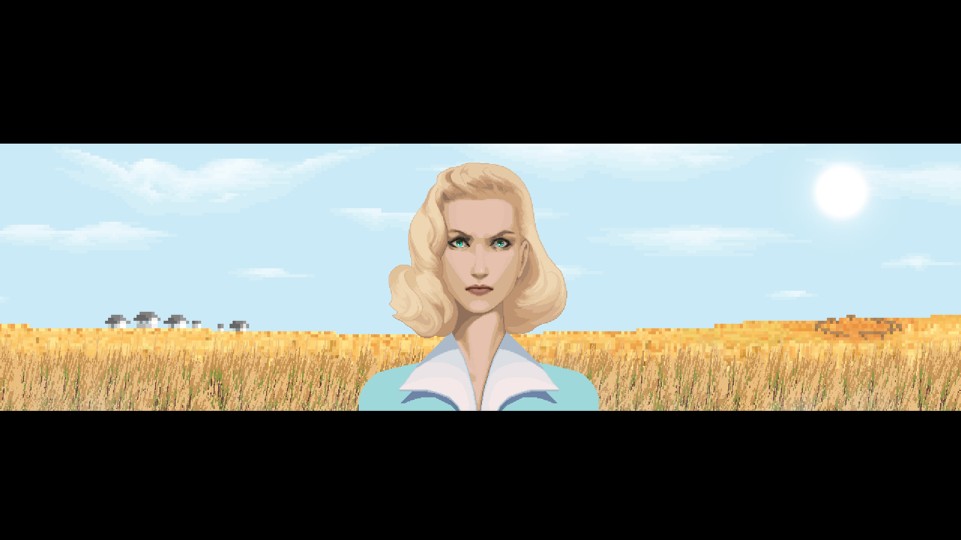
I write with the assumption that everyone would look up the content warnings for a particular media I mention themselves, but I don’t think I’ve ever made that clear. So here is a good opportunity to do so, because Loretta is a lot. I can’t say it shocked me, but there were plenty of oof >_< moments. It specifically mentions that it tries to be honest about its subject matter and time period without shying away from the bad, but it doesn’t do that for shock value alone and with enough modern sensibility.